Prime Numbers
You can easily arrange balls in three rows and four columns
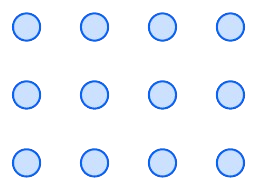
This worked because . In other words, because can be represented as a product of two smaller numbers, and .
The question to ask is: "How many groups of apples are possible?"
Note that we do not include trivial decompositions like (a rectangle with one row or one column).
On the other hand, does not have any non-trivial decompositions (only and ), meaning it is prime.
Definition
A prime number is a positive integer that cannot be represented as the product of two smaller positive integers.
A number is prime if it does not have positive integer divisors except for and .
Indeed, if is composite where and are non-trivial divisors, then and are both smaller than , making it impossible for either one to be equal to . And if a number has some non-trivial divisor , then for some positive integer we must have by the definition of a divisor, and neither nor can be equal to , so both must be smaller than .
Naive Approach - Trial Divison
The most straightforward method to determine if a number is prime is through trial division. We divide the number by all integers from up to its square root. If none of these divisions result in a remainder of , the number is prime.
def is_prime_naive(num):
"""Checks if a number is prime using trial division."""
if num <= 1:
return False
for i in range(2, int(num**0.5) + 1):
if num % i == 0:
return False
return True
The provided Python function, is_prime_naive, efficiently determines whether a given integer is a prime number. It first handles the base case where the number is less than or equal to , which is not prime. The function then iterates through potential divisors from up to the square root of the number.
If any divisor divides the number evenly, it's not prime and the function returns False. If the loop completes without finding a divisor, the number must be prime, and the function returns True. This optimization of checking only up to the square root is based on the mathematical property that if a number has a divisor greater than its square root, it must also have a corresponding divisor less than its square root.